Table of Contents
Destructor is a special method that is called when an object gets destroyed. On the other hand, a constructor is used to create and initialize an object of a class.
After reading this article, you will learn:
- How create a destructor in Python
- The use of
__del__()
method - Wokring of a destructor
What is Destructor in Python?
In object-oriented programming, A destructor is called when an object is deleted or destroyed. Destructor is used to perform the clean-up activity before destroying the object, such as closing database connections or filehandle.
Python has a garbage collector that handles memory management automatically. For example, it cleans up the memory when an object goes out of scope.
But it’s not just memory that has to be freed when an object is destroyed. We must release or close the other resources object were using, such as open files, database connections, cleaning up the buffer or cache. To perform all those cleanup tasks we use destructor in Python.
The destructor is the reverse of the constructor. The constructor is used to initialize objects, while the destructor is used to delete or destroy the object that releases the resource occupied by the object.
In Python, destructor is not called manually but completely automatic. destructor gets called in the following two cases
- When an object goes out of scope or
- The reference counter of the object reaches 0.
“Ready to take your python skills to the next level? Sign up for a free demo today!”
In Python, The special method __del__()
is used to define a destructor. For example, when we execute del object_name
destructor gets called automatically and the object gets garbage collected.
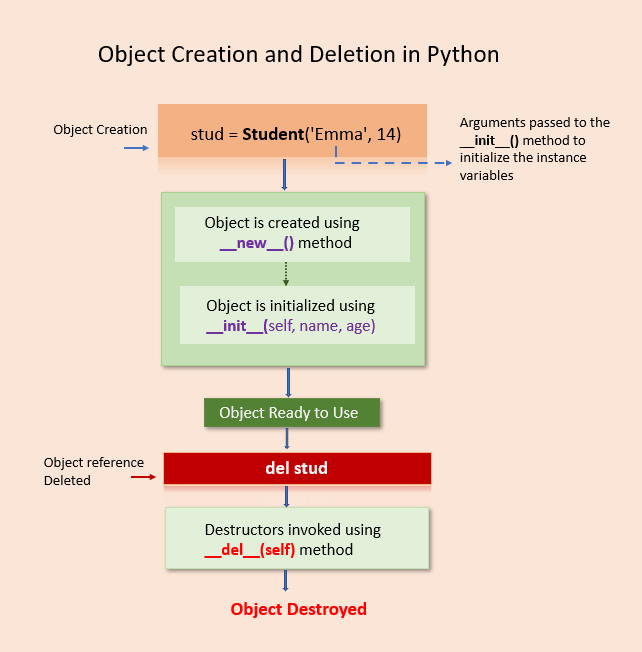
Create Destructor using the __del__()
Method
The magic method __del__()
is used as the destructor in Python. The __del__()
method will be implicitly invoked when all references to the object have been deleted, i.e., is when an object is eligible for the garbage collector.
This method is automatically called by Python when the instance is about to be destroyed. It is also called a finalizer or (improperly) a destructor.
Syntax of destructor declaration
Where,
def
: The keyword is used to define a method.__del__()
Method: It is a reserved method. This method gets called as soon as all references to the object have been deletedself
: The first argumentself
refers to the current object.
Note: The __del__()
method arguments are optional. We can define a destructor with any number of arguments.
Example
Let’s see how to create a destructor in Python with a simple example. In this example, we’ll create a Class Student with a destructor. We’ll see: –
- How to implement a destructor
- how destructor gets executed when we delete the object.
Output
Inside Constructor Object initialized Hello, my name is Emma Inside destructor Object destroyed
Note:
As you can see in the output, the __del__()
method get called automatically is called when we deleted the object reference using del s1
.
The destructor has called when the reference to the object is deleted or the reference count for the object becomes zero
“Experience the power of our web development course with a free demo – enroll now!”
Important Points to Remember about Destructor
- The
__del__
method is called for any object when the reference count for that object becomes zero. - The reference count for that object becomes zero when the application ends, or we delete all references manually using the
del
keyword. - The destructor will not invoke when we delete object reference. It will only invoke when all references to the objects get deleted.
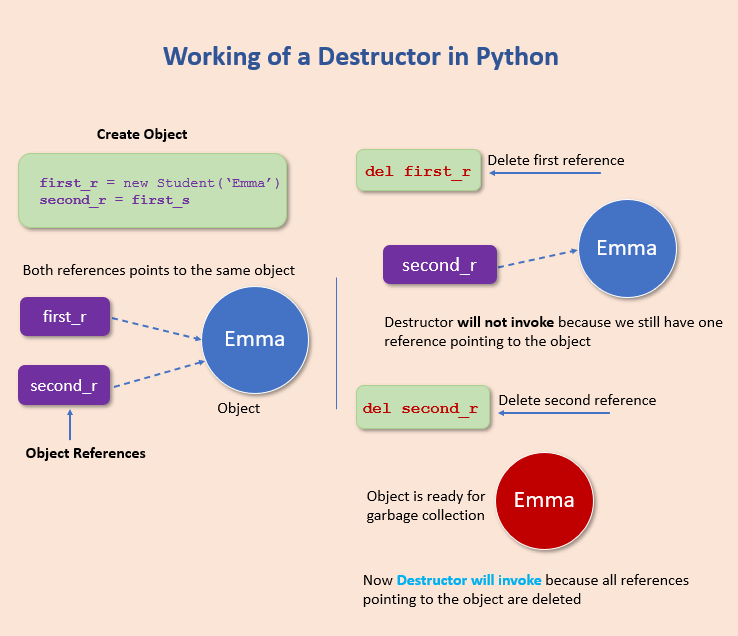
Example:
Let’s understand the above points using the example.
- First create object of a student class using
s1 = student('Emma')
- Next, create a new object reference
s2
by assignings1
tos2
usings2=s1
- Now, both reference variables
s1
ands2
point to the same object. - Next, we deleted reference
s1
- Next, we have added 5 seconds of sleep to the main thread to understand that destructors only invoke when all references to the objects get deleted.
Output:
Inside Constructor Hello, my name is Emma
After Sleep
After sleep
Hello, my name is Emma
Object destroyed
- As you can see in the output destructors only invoked when all references to the objects get deleted.
- Also, the destructor is executed when the code (application) ends and the object is available for the garbage collector. (I.e., we didn’t delete object reference
s2
manually usingdel s2
).
Cases when Destructor doesn’t work Correctly
The __del__
is not a perfect solution to clean up a Python object when it is no longer required. In Python, the destructor behave behaves weirdly and doesn’t execute in the following two cases.
- Circular referencing when two objects refer to each other
- Exception occured in __init__() method
“Get hands-on with our python course – sign up for a free demo!”
Circular Referencing
The __del()__()
doesn’t work correctly in the case of circular referencing. In circular referencing occurs when two objects refer to each other.
When both objects go out of scope, Python doesn’t know which object to destroy first. So, to avoid any errors, it doesn’t destroy any of them.
In short, it means that the garbage collector does not know the order in which the object should be destroyed, so it doesn’t delete them from memory.
Ideally, the destructor must execute when an object goes out of scope, or its reference count reaches zero.
But the objects involved in this circular reference will remain stored in the memory as long as the application will run.
Example:
In the below example, ideally, both Vehicle and Car objects must be destroyed by the garbage collector after they go out of scope. Still, because of the circular reference, they remain in memory.
I’d recommend using Python’s with statement for managing resources that need to be cleaned up.
Output:
Vehicle 12 created Car 12 created
Exception in __init__
Method
In object-oriented programming, A constructor is a special method used to create and initialize an object of a class. using the __init__() method we can implement a constructor to initialize the object.
In OOP, if any exception occurs in the constructor while initializing the object, the constructor destroys the object.
Likewise, in Python, if any exception occurs in the init method while initializing the object, the method del gets called. But actually, an object is not created successfully, and resources are not allocated to it
even though the object was never initialized correctly, the del method will try to empty all the resources and, in turn, may lead to another exception.
Example:
Output:
Traceback (most recent call last): Release resources Exception: Not Allowed
Conclusion
- In object-oriented programming, A destructor is called when an object is deleted or destroyed.
- Destructor is used to perform the clean-up activity before destroying the object, such as closing database connections or filehandle.
- In Python we use
__del__()
method to perform clean-up task before deleting the object. - The destructor will not invoke when we delete object reference. It will only invoke when all references to the objects get deleted.